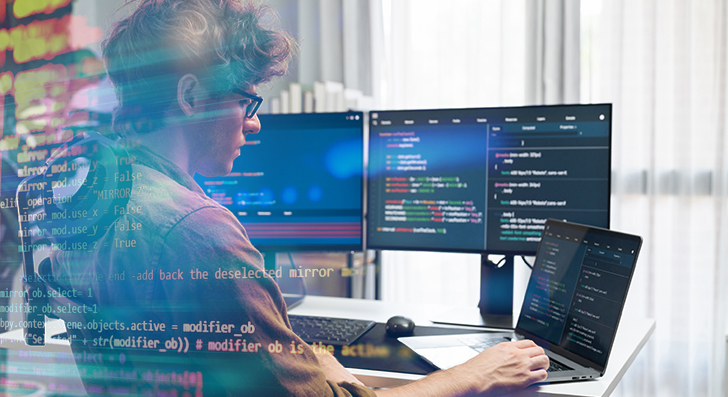
Scalability suggests your application can manage development—more people, far more information, and much more traffic—with no breaking. As being a developer, building with scalability in your mind saves time and stress later on. Right here’s a transparent and useful guidebook that will help you get started by Gustavo Woltmann.
Layout for Scalability from the Start
Scalability just isn't some thing you bolt on afterwards—it should be aspect of one's program from the start. Several purposes fall short when they increase quick mainly because the original design can’t take care of the additional load. Like a developer, you might want to Feel early regarding how your method will behave stressed.
Get started by developing your architecture to become versatile. Stay clear of monolithic codebases in which all the things is tightly connected. Alternatively, use modular structure or microservices. These patterns split your application into smaller sized, impartial sections. Every module or provider can scale By itself without affecting The full process.
Also, think of your databases from working day one. Will it want to manage one million buyers or simply a hundred? Select the right kind—relational or NoSQL—determined by how your facts will mature. Strategy for sharding, indexing, and backups early, even if you don’t need them but.
A different vital place is to stay away from hardcoding assumptions. Don’t write code that only functions beneath present-day conditions. Consider what would come about If the user base doubled tomorrow. Would your application crash? Would the databases decelerate?
Use style designs that guidance scaling, like message queues or function-driven units. These help your app deal with a lot more requests devoid of having overloaded.
Whenever you Develop with scalability in mind, you're not just getting ready for success—you are decreasing future headaches. A well-prepared technique is easier to maintain, adapt, and mature. It’s better to prepare early than to rebuild afterwards.
Use the ideal Databases
Selecting the correct databases is often a essential A part of constructing scalable applications. Not all databases are crafted the exact same, and using the Incorrect you can sluggish you down or perhaps cause failures as your application grows.
Commence by comprehension your knowledge. Is it remarkably structured, like rows within a table? If Of course, a relational database like PostgreSQL or MySQL is a superb in shape. They're potent with interactions, transactions, and consistency. In addition they assist scaling methods like browse replicas, indexing, and partitioning to deal with extra targeted traffic and data.
If the facts is more versatile—like person action logs, products catalogs, or documents—look at a NoSQL choice like MongoDB, Cassandra, or DynamoDB. NoSQL databases are greater at handling huge volumes of unstructured or semi-structured details and might scale horizontally more simply.
Also, consider your go through and produce patterns. Do you think you're accomplishing plenty of reads with less writes? Use caching and skim replicas. Have you been managing a heavy compose load? Check into databases that can manage significant create throughput, as well as party-based details storage systems like Apache Kafka (for short-term knowledge streams).
It’s also clever to think forward. You may not will need advanced scaling attributes now, but selecting a database that supports them means you won’t require to change later on.
Use indexing to speed up queries. Keep away from avoidable joins. Normalize or denormalize your data according to your entry designs. And generally keep track of database efficiency while you expand.
In a nutshell, the best database is determined by your app’s construction, pace requirements, And just how you be expecting it to increase. Just take time to choose properly—it’ll conserve lots of difficulty later.
Improve Code and Queries
Speedy code is vital to scalability. As your application grows, just about every modest hold off adds up. Poorly penned code or unoptimized queries can decelerate functionality and overload your program. That’s why it’s vital that you Develop efficient logic from the start.
Get started by producing clear, easy code. Avoid repeating logic and take away nearly anything unwanted. Don’t select the most intricate Resolution if an easy a single will work. Maintain your capabilities limited, targeted, and straightforward to test. Use profiling tools to search out bottlenecks—spots in which your code takes much too prolonged to run or uses an excessive amount memory.
Up coming, look at your databases queries. These often sluggish issues down in excess of the code itself. Ensure that Every question only asks for the information you truly require. Stay clear of Pick *, which fetches all the things, and as an alternative find certain fields. Use indexes to hurry up lookups. And avoid undertaking a lot of joins, Specifically throughout huge tables.
For those who discover the exact same data getting asked for again and again, use caching. Retailer the final results quickly making use of instruments like Redis or Memcached so you don’t really have to repeat costly operations.
Also, batch your databases functions after you can. Rather than updating a row one by one, update them in teams. This cuts down on overhead and will make your application much more productive.
Make sure to take a look at with big datasets. Code and queries that operate high-quality with a hundred documents may crash after they have to deal with 1 million.
In a nutshell, scalable applications are rapid applications. Keep the code limited, your queries lean, and use caching when required. These measures support your application remain clean and responsive, whilst the load will increase.
Leverage Load Balancing and Caching
As your app grows, it's to deal with far more people plus more targeted visitors. If everything goes by means of a single server, it's going to swiftly become a bottleneck. That’s exactly where load balancing and caching come in. Both of these applications assistance keep the app quickly, stable, and scalable.
Load balancing spreads incoming traffic throughout numerous servers. Instead of a person server accomplishing the many get the job done, the load balancer routes end users to distinct servers depending on availability. This means no one server will get overloaded. If one particular server goes down, the load balancer can deliver visitors to the Some others. Applications like Nginx, HAProxy, or cloud-dependent remedies from AWS and Google Cloud make this simple to set up.
Caching is about storing facts briefly so it can be reused immediately. When end users request a similar facts once more—like an item website page or maybe a profile—you don’t must fetch it from the databases each time. You could serve it from the cache.
There are 2 common sorts of caching:
1. Server-aspect caching (like Redis or Memcached) shops facts in memory for quickly obtain.
2. Client-aspect caching (like browser caching or CDN caching) stores static documents close to the consumer.
Caching cuts down database load, increases speed, and can make your app a lot more economical.
Use caching for things that don’t transform frequently. And always be sure your cache is current when info does transform.
In brief, load balancing and caching are basic but powerful equipment. Alongside one another, they help your app cope with more consumers, continue to be fast, and Recuperate from difficulties. If you intend to mature, you'll need equally.
Use Cloud and Container Applications
To build scalable programs, you may need instruments that permit your app develop very easily. That’s wherever cloud platforms and containers are available. They give you flexibility, minimize set up time, and make scaling A great deal smoother.
Cloud platforms like Amazon Internet Providers (AWS), Google Cloud Platform (GCP), and Microsoft Azure Allow you to lease servers and companies as you would like them. You don’t really have to buy hardware or guess long term capacity. When targeted traffic will increase, you may insert extra sources with only a few clicks or instantly here utilizing auto-scaling. When visitors drops, you are able to scale down to save money.
These platforms also provide providers like managed databases, storage, load balancing, and safety resources. You are able to concentrate on developing your app instead of running infrastructure.
Containers are A further important tool. A container offers your application and almost everything it has to run—code, libraries, configurations—into just one device. This makes it simple to maneuver your application among environments, from your notebook on the cloud, without having surprises. Docker is the most popular tool for this.
When your application employs several containers, tools like Kubernetes assist you deal with them. Kubernetes handles deployment, scaling, and recovery. If a person portion of one's application crashes, it restarts it instantly.
Containers also make it straightforward to independent parts of your application into solutions. You could update or scale areas independently, which can be perfect for functionality and reliability.
Briefly, utilizing cloud and container applications implies you could scale quickly, deploy easily, and Recuperate immediately when difficulties materialize. If you'd like your application to develop devoid of limits, start off using these resources early. They save time, minimize hazard, and assist you to keep centered on developing, not repairing.
Observe Every thing
In case you don’t monitor your application, you gained’t know when matters go Incorrect. Monitoring can help the thing is how your app is executing, place challenges early, and make much better conclusions as your application grows. It’s a important Portion of making scalable units.
Begin by tracking simple metrics like CPU utilization, memory, disk Place, and reaction time. These show you how your servers and services are performing. Equipment like Prometheus, Grafana, Datadog, or New Relic can assist you accumulate and visualize this details.
Don’t just monitor your servers—keep track of your app as well. Keep watch over just how long it's going to take for buyers to load internet pages, how frequently faults happen, and where they occur. Logging tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can help you see what’s happening inside your code.
Set up alerts for important problems. For example, In case your response time goes higher than a Restrict or maybe a assistance goes down, it is best to get notified quickly. This will help you resolve difficulties rapidly, typically just before customers even notice.
Checking can be beneficial whenever you make changes. For those who deploy a different attribute and see a spike in faults or slowdowns, you may roll it back before it will cause true harm.
As your application grows, targeted traffic and information maximize. With no monitoring, you’ll miss indications of problems until it’s far too late. But with the proper applications in position, you stay on top of things.
In short, checking helps you maintain your app trusted and scalable. It’s not nearly recognizing failures—it’s about comprehending your procedure and ensuring it really works nicely, even stressed.
Final Feelings
Scalability isn’t only for huge companies. Even modest applications have to have a powerful Basis. By designing meticulously, optimizing wisely, and using the ideal resources, you could Develop applications that grow easily without the need of breaking under pressure. Start off compact, Feel major, and build wise.